title: swan.showToast header: develop nav: api sidebar: toast_swan-showToast
webUrl: https://qft12m.smartapps.cn/swan-api/toast/toast
解释:显示消息提示框
方法参数
Object object
object
参数说明
属性名 | 类型 | 必填 | 默认值 | 说明 |
---|---|---|---|---|
title | String | 是 | 提示的内容 | |
icon | String | 否 | success | 图标,有效值"success"、"loading"、"none" 。 |
image | String | 否 | 自定义图标的本地路径,image 的优先级高于 icon | |
duration | Number | 否 | 2000 | 提示的延迟时间,单位毫秒。 |
success | Function | 否 | 接口调用成功的回调函数 | |
fail | Function | 否 | 接口调用失败的回调函数 | |
complete | Function | 否 | 接口调用结束的回调函数(调用成功、失败都会执行) | |
mask | Boolean | 否 | false | 是否显示透明蒙层,防止触摸穿透。 |
icon有效值
有效值 | 说明 |
---|---|
success | 显示成功图标,此时 title 文本最多显示 7 个汉字长度。默认值 |
loading | 显示加载图标,此时 title 文本最多显示 7 个汉字长度。 |
none | 不显示图标,此时 title 文本最多可显示两行。 |
示例
扫码体验
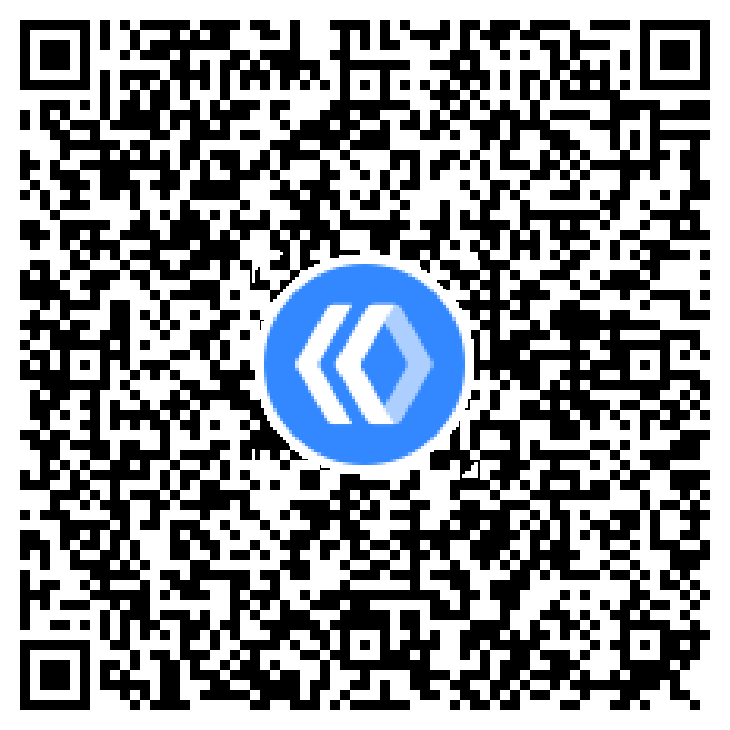
代码示例1 - 默认样式
:::codeTab
<view class="card-area">
<view class="top-description border-bottom">默认样式</view>
<button bindtap="showToast" type="primary" hover-stop-propagation="true">默认toast</button>
</view>
Page({
showToast() {
swan.showToast({
title: '默认toast',
mask: false,
success: res => {
this.setData({'disabled': false});
},
fail: err => {
console.log('showToast fail', err);
}
});
}
});
代码示例2 - 无图标toast
:::codeTab
<view class="card-area">
<view class="top-description border-bottom">
<view>设置不显示图标</view>
<view>icon: 'none'</view>
</view>
<button bindtap="showToastIcon" type="primary" hover-stop-propagation="true">无图标toast</button>
</view>
Page({
showToastIcon() {
swan.showToast({
title: '单行最多十四个文字单行最多十四个文字',
icon: 'none',
mask: false,
success: res => {
this.setData({'disabled': false});
},
fail: err => {
console.log('showToast fail', err);
}
});
}
});
代码示例3 - 显示loading图标
:::codeTab
<view class="card-area">
<view class="top-description border-bottom">
<view>设置显示loading图标</view>
<view>icon: 'loading'</view>
</view>
<button bindtap="showToastLoading" type="primary" hover-stop-propagation="true">loading toast</button>
</view>
Page({
showToastLoading() {
swan.showToast({
mask: true,
title: '正在加载...',
icon: 'loading',
mask: false,
success: res => {
this.setData({'disabled': false});
},
fail: err => {
console.log('showToast fail', err);
}
});
}
});
代码示例4 - 延迟5000毫秒的toast
:::codeTab
<view class="card-area">
<view class="top-description border-bottom">
<view>设置延迟时间</view>
<view>duration: 5000</view>
</view>
<button bindtap="showToastDuration" type="primary" hover-stop-propagation="true">延迟5000毫秒的toast</button>
</view>
Page({
showToastDuration() {
swan.showToast({
duration: 5000,
title: '5000毫秒后隐藏',
icon: 'none',
mask: false,
success: res => {
this.setData({'disabled': false});
},
fail: err => {
console.log('showToast fail', err);
}
});
}
});
代码示例5 - 隐藏toast
:::codeTab
<view class="card-area">
<view class="top-description border-bottom">隐藏toast</view>
<button bindtap="hideToast" type="primary" disabled="{{disabled}}" hover-stop-propagation="true">隐藏toast</button>
</view>
Page({
hideToast() {
swan.hideToast();
swan.hideLoading();
}
});
参考示例
:::
参考示例1 - 开发者可自定义showToast样式
:::codeTab
<view class="wrap">
<button type="primary" bindtap="clickbtn"> 点击 </button>
<view animation="{{animationData}}" class="toast-view" s-if="{{showModalStatus}}">要显示的内容
</view>
</view>
Page({
data: {
animationData: "",
showModalStatus: false
},
showModal() {
var animation = swan.createAnimation({
duration: 200,
timingFunction: "linear",
delay: 0
})
animation.translateY(200).step()
this.setData({
animationData: animation.export(),
showModalStatus: true
})
let that = this;
setTimeout(function () {
animation.translateY(0).step()
that.setData({
animationData: animation.export()
})
}, 200)
setTimeout(function () {
if(that.data.showModalStatus){
that.hideModal();
}
}, 5000)
},
clickbtn() {
if(this.data.showModalStatus){
this.hideModal();
}
else {
this.showModal();
}
},
hideModal() {
this.setData({
showModalStatus: false
})
},
})
:::
参考示例2 - showModal和showToast是否可共存
:::codeTab
<view class="container">
<view>
<view class="card-area">
<view class="top-description border-bottom">showModal和showToast可共存</view>
<button data-title="success" data-icon="success" bindtap="showToast" type="primary" hover-stop-propagation="true">点击弹出toast</button>
<button data-title="loading" data-icon="loading" bindtap="showModal" type="primary" hover-stop-propagation="true">点击弹出modal</button>
</view>
</view>
</view>
Page({
data: { },
showToast(e) {
this.toast(e.currentTarget.dataset.title, e.currentTarget.dataset.icon);
},
toast(title, icon) {
swan.showToast({
title,
icon,
mask: false, // 此属性设置为true不能打断toast
success: res => {
console.log('showToast success', res);
},
fail: err => {
console.log('showToast fail', err);
}
})
},
showModal(){
swan.showModal({
title: 'title',
content: 'content'
});
}
});
:::
错误码
Android
错误码 | 说明 |
---|---|
202 | 解析失败,请检查参数是否正确 |
302 | 找不到调起协议对应端能力方法 |
iOS
错误码 | 说明 |
---|---|
202 | 解析失败,请检查参数是否正确 |
Bug&Tip
- Tip:swan.showLoading 和 swan.showToast 同时只能显示一个。
- Tip:swan.showToast 应与 swan.hideToast 配对使用。