String类
基础概念
- jeeString类又称作不可变字符序列
- String类位于java.lang包中,java程序默认导入java.lang包下的所有类
- Java字符串就是Unicode字符序列,例如字符串”Java”就是4个Unicode字符”J”、”a”、”v”、”a”组成的
- Java没有内置的字符串类型,而是在标准Java类库中提供了一个预定义的类String,每个双引号扩展起来的字符串都是String类的一个实例
- 双引号引起来的字符串均存放在字符串常量池中 ```java String s1 = “abc”; // 字符串常量,放置于字符串常量池 String s2 = new String(“abc”); // 建立一个新的字符串对象 String s3 = “abc”; String s3 = “aBC”;
System.out.println(s1 == s2); // false System.out.println(s2 == s3); // true System.out.println(s1.equals(s3)); // true 判断字符串的值是否相等 System.out.println(s1.equalsIgnoreCase(s4)); // true 判断字符串忽略大小写值相等
<a name="tozJM"></a>
## String类方法列表
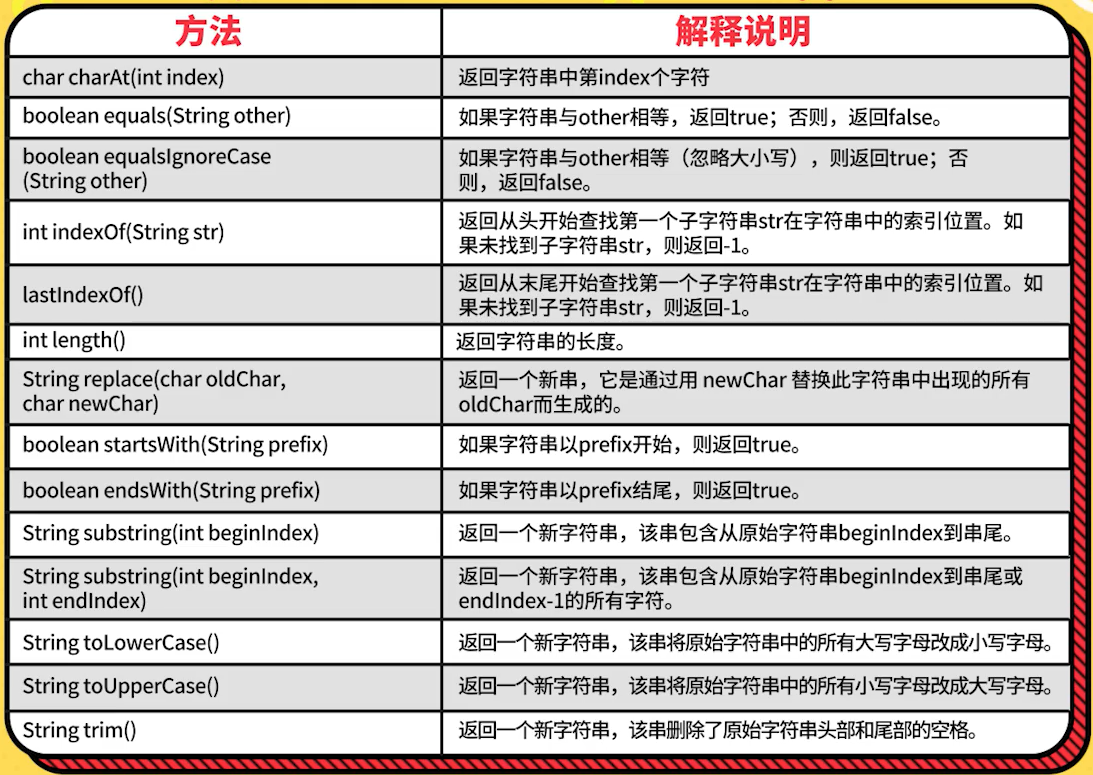
<a name="w51Pw"></a>
# StringBuffer、StringBuilder类
<a name="Lpg9w"></a>
## 基础概念
| **String** | **StringBuffer** | **StringBuilder** |
| --- | --- | --- |
| 不可变字符序列 | 可变字符序列 | 可变字符序列 |
| | 线程安全,做线程同步检查,效率较低 | 线程不安全,不做线程同步检查,因此效率高。建议使用该类。 |
<a name="I8GwU"></a>
## 常用方法
- StringBuilder与StringBuffer的常用方法是一致的
- append("a"),追加
- insert(0,"a"),插入
- delete(0,2),删除
- deleteCharAt(0),删除
- reverse(),逆序
<a name="vbvyV"></a>
# 包装类
<a name="QyTBr"></a>
## 八种基本数据类型的包装类
| 基本数据类型 | byte | boolean | short | char | int | long | float | double |
| --- | --- | --- | --- | --- | --- | --- | --- | --- |
| 包装类 | Byte | Boolean | Short | Char | **Integer** | Long | Float | Double |
<a name="Wbbyw"></a>
## 包装类的使用方式
```java
// 基本数据类型转化成Integer对象
Integer int1 = Integer.valueOf(100);
// 包装类对象转成基本数据类型
int int2 = int1.intValue();
long long1 = int1.longValue();
// 字符串转Integer对象
Integer int3 = Integer.parseInt("321");
// 类型最大数
System.out.println("int能表示的最大整数:" + Integer.MAX_VALUE);
自动装箱和自动拆箱
Integer a = 100; // 自动装箱,编译器完成正常类型转换操作
int b = a; // 自动拆箱
整型、char类型所对应的包装类在自动装箱时,对于-128到127之间的值会进行缓存处理,以提高效率
Integer a = 100;
Integer b = Integer.valueOf(100);
System.out.println(a == b); // true a b 是同一个类,由IntegerCache类处理
Integer c = 300;
Integer d = Integer.valueOf(300);
System.out.println(c == d); // false c d 不是一个类
源码解读Integer类
```java public class MyInteger { public static final int LOW_VALUE = -128; public static final int MAX_VALUE = 127;
private int value; private static MyInteger[] integerCache = new MyInteger[256];
static {
for (int i = MyInteger.LOW_VALUE; i <= MyInteger.MAX_VALUE; i++) {
integerCache[i + (-MyInteger.LOW_VALUE)] = new MyInteger(i);
}
}
public static MyInteger valueOf(int val){
if(val>= MyInteger.LOW_VALUE && val<= MyInteger.MAX_VALUE){
return integerCache[val + (-MyInteger.LOW_VALUE)];
}else {
return new MyInteger(val);
}
}
public int intValue(){
return value;
}
public String toString(){
return value + "";
}
private MyInteger(int val){
value = val;
}
public static void main(String[] args) {
MyInteger a = MyInteger.valueOf(100);
MyInteger b = MyInteger.valueOf(100);
System.out.println(a == b);
MyInteger c = MyInteger.valueOf(300);
MyInteger d = MyInteger.valueOf(300);
System.out.println(c == d);
}
}
<a name="H9uE9"></a>
# 时间相关类
<a name="XuNJG"></a>
## Date
```java
public static void testDate(){
// 返回当前毫秒数
long nowMillis = System.currentTimeMillis();
Date d1 = new Date();
Date d2 = new Date(1000L * 3600 * 24 * 100);
}
DateFormat
时间和字符串之间互相转换
public static void testDateFormat(){
// 日期对象转字符串
DateFormat df = new SimpleDateFormat("yyyy-MM-dd hh:mm:ss");
Date d2 = new Date(1000L * 3600 * 24 * 100);
Sting strD2 = df.format(d2);
// 字符串转日期对象
Sting strD3 = "2049-10-1 10:10:20";
Date d3 = df.parse(str3);
// 获取当前时间是当年的第几天
Dateformat df2 = new SimpleDateFormat("D");
String theDay = df2.format(d2);
}
Calendar
public static void testCalendar(){
// 月份 0-11一月到十二月 星期1-7 周日到周六
Calendar calendar = new GregorianCalendar(1989,3,14,8,8,8);
int year = calendar.get(Calendar.YEAR);
int month = calendar.get(Calendar.MONTH)
calendar.set(Calendar.YEAR,2017)
// 返回的日期对象
Date d1 = calendar.getTime();
// 返回的毫米数
long millis1 = calendar.getTimeInMillis();
// 日期计算,增加1000天
calendar.add(Calendar.DATE,1000)
// 日期计算,减少30年
calendar.add(Calendar.YEAR,30)
}